The Collections framework in Java
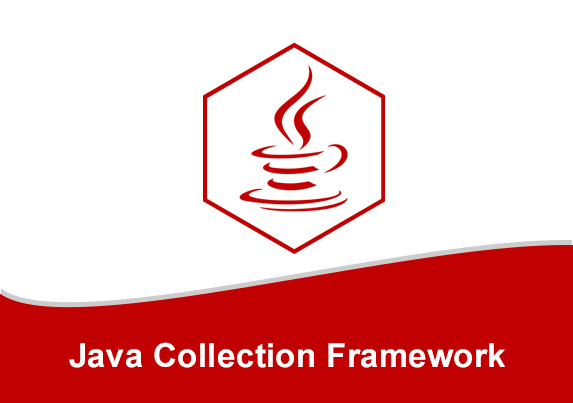
Today’s topic is a little bit technical as it is related to Java developers. We are going to speak about Java and its frameworks like Collections, the Map interface, Java TreeMap class, etc. Our team continues to study interesting and essential features in web and mobile development, so let’s continue!
Basic concepts
Collections in java is a framework that provides an architecture to store and manipulate the group of objects. The Java Collection Framework is divided into two interfaces: Collection and Map. These interfaces isolate all collections that enter the two types of data boxes: simple sets of elements and sets of “key”. Java Collection simply means a single unit of objects. Java Collection framework provides many interfaces (Set, List, Queue, Deque etc.) and classes (ArrayList, Vector, LinkedList, PriorityQueue, HashSet, LinkedHashSet, TreeSet etc).
Methods of Collection interface
-
- boolean add(Object element) is used to insert an element in this collection.
- boolean addAll(Collection c) is used to insert the specified collection elements in the invoking collection.
- boolean remove(Object element) is used to delete an element from this collection.
- boolean removeAll(Collection c) is used to delete all the elements of specified collection from the invoking collection.
- boolean retainAll(Collection c) is used to delete all the elements of invoking collection except the specified collection.
- int size() return the total number of elements in the collection.
- void clear() removes the total no of element from the collection.
- boolean contains(Object element) is used to search an element.
- boolean containsAll(Collection c) is used to search the specified collection in this collection.
- Iterator iterator() returns an iterator.
- Object[] toArray() converts collection into array.
- boolean isEmpty() checks if collection is empty.
- boolean equals(Object element) matches two collection.
- int hashCode() returns the hashcode number for collection.
How ArrayList Works Internally in Java
ArrayList is a resizable array implementation of the List interface i.e. ArrayList grows dynamically as the elements are added to it.
If the size of the current elements (including the new element to be added to the ArrayList) is greater than the maximum size of the array then increase the size of array. But the size of the array can not be increased dynamically. So, what happens internally is, a new Array is created and the old array is copied into the new array.
Java LinkedList class
Java LinkedList class uses doubly linked list to store the elements. It provides a linked-list data structure. It inherits the AbstractList class and implements List and Deque interfaces. The important points about Java LinkedList are:
- Java LinkedList class can contain duplicate elements.
- Java LinkedList class maintains insertion order.
- Java LinkedList class is non synchronized.
- In Java LinkedList class, manipulation is fast because no shifting needs to be occurred.
- Java LinkedList class can be used as list, stack or queue.
Doubly Linked List
In case of doubly linked list, we can add or remove elements from both side.
The Map Interface
A Map is an object that maps keys to values. A map cannot contain duplicate keys: Each key can map to at most one value. It models the mathematical function abstraction. The Map interface includes methods for basic operations (such as put, get, remove, containsKey, containsValue, size, and empty), bulk operations (such as putAll and clear), and collection views (such as keySet, entrySet, and values).The Java platform contains three general-purpose Map implementations: HashMap, TreeMap, and LinkedHashMap. Their behavior and performance are precisely analogous to HashSet, TreeSet, and LinkedHashSet, as described in The Set Interface section.
Java TreeMap class
Java TreeMap class implements the Map interface by using a tree. It provides an efficient means of storing key/value pairs in sorted order.
The important points about Java TreeMap class are:
- A TreeMap contains values based on the key. It implements the NavigableMap interface and extends AbstractMap class.
- It contains only unique elements.
- It cannot have null key but can have multiple null values.
- It is same as HashMap instead maintains ascending order.